Table Of Content
Discover How You Can Use SQL to Manage Your Data Effectively
SQL is a powerful programming language that you can use for a variety of tasks, from creating and reading tables to data analysis. In this article, we shall explore and learn about all the things you can do with SQL and how you can effectively use it.
We will start by discussing what SQL is and its uses. Then we will cover the basics of SQL, including how to create, read, and update tables. Next, we will move on to more advanced topics, such as joins, subqueries, and window functions.
Finally, we will provide tips for improving your SQL skills and show you how to avoid common mistakes. With this guide, you will develop an understanding of SQL for everything from data entry to complex analysis.
What Does SQL Stand for And What is SQL Database?
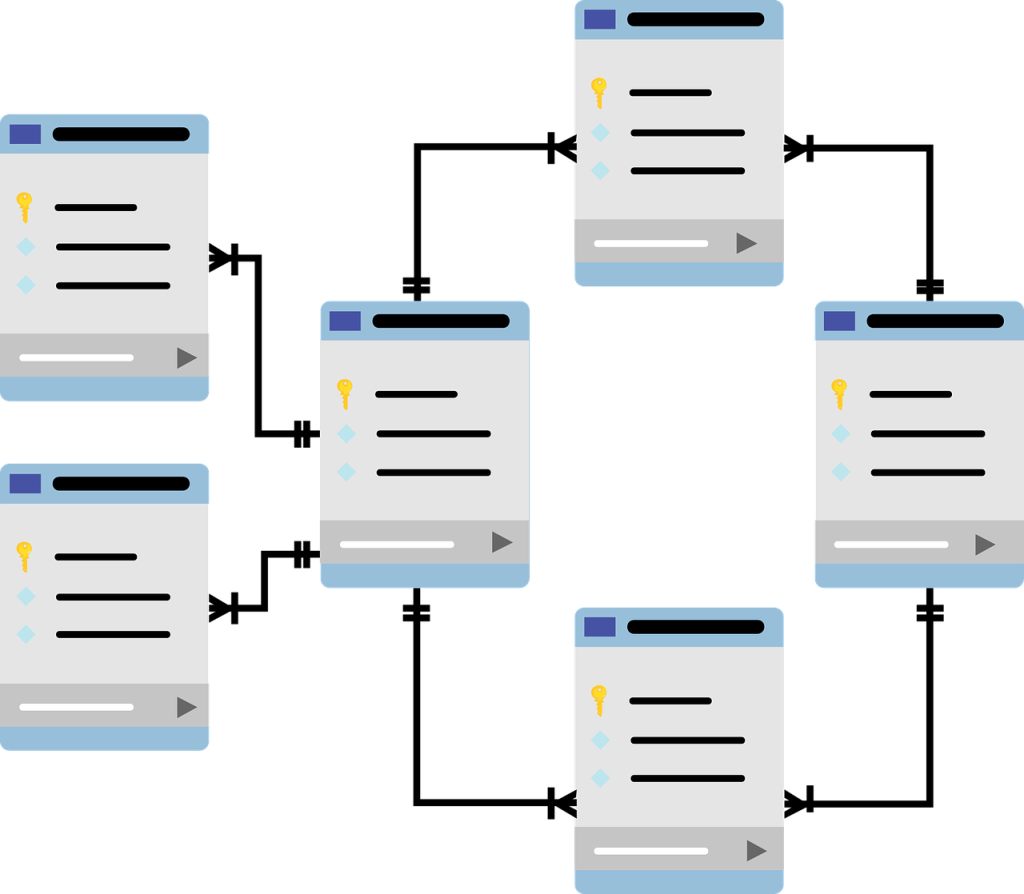
SQL (Structured Query Language) is a powerful tool that enables a user to communicate with and manipulate data for relational databases; these SQL databases are used to store structured data in a collection of tables.
A variety of tasks are performed with the help of SQL, from creating, reading and updating tables to data analysis. It works as a standard for all major database vendors, including Microsoft SQL Server, Oracle, MySQL, and PostgreSQL. This means that once you learn SQL, you can use it with any database system.
Being a declarative language, in using SQL, all you need to do is specify what you want to achieve rather than how to achieve it. This makes it easy to learn and use it, but mastering it requires a long time, and continuous practice. That is often why many businesses prefer using custom database development services to create their company’s official database.
What is SQL Used For?
SQL can be used for many applications, some of these are:
- Developers and database administrators use SQL to write Data Integration Scripts.
- Because SQL is semantically easy, data analysts use it for storing and accessing large amounts of data. This helps them overcome the hassle of copying data onto other applications.
- Data analysts also use SQL because auditing is easier with it, as compared to spreadsheet tools.
- Used for easily modifying and manipulating date and database tables.
- The knowledge of different SQL and SQL database concepts equips QA software testers with the ability to effectively perform database testing. Therefore, another major use-case for SQL is that it helps Quality Assurance Officers as one of their popular software testing tools.
What Are The Benefits of Using SQL?
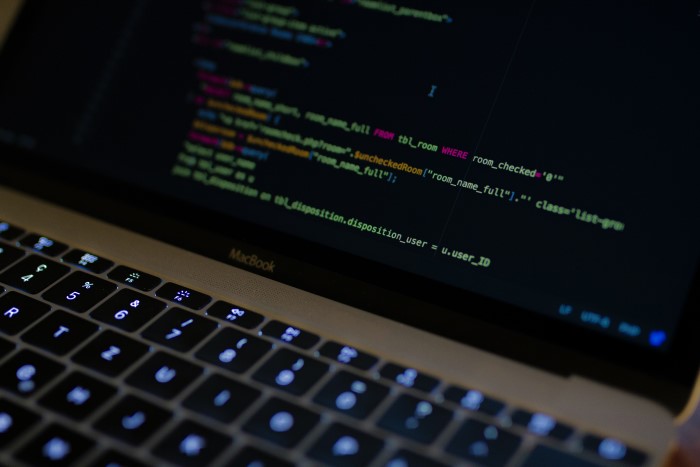
The benefits of using SQL are as followed:
- Standardized syntax: SQL has been around for years which is why it’s well documented and provides all its user base with a uniform platform worldwide.
- Ease of use & Powerful Performance: SQL is a declarative language, which makes it easy to learn and use. Moreover, answers for complex queries or large amounts of data can be retrieved quickly and efficiently. Which means SQL helps massively cut down wait times, when inserting, deleting and manipulating data.
- Flexibility: SQL has the added advantage of flexibility which allows it to function on any operating system. Also, if need be, it can be embedded into different applications as per our requirement and usage.
- Low Code Friendly: The best part about SQL is that data retrieval does not require users to write lengthy lines of code. The keywords are very basic, such as SELECT, UPDATE, etc., and the syntactic rules are simple in SQL, which is why it’s known as a user-friendly language.
What Are The Basics of SQL?
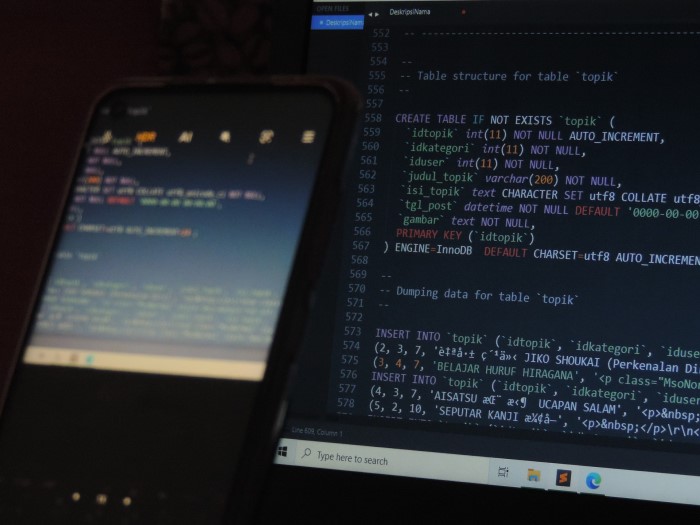
Now that we have covered what SQL is, let ‘s take a look at the basics of how to use it. You can use it for a lot of different data related tasks, creating new tables, processing the data, and even migrating an existing database to a new host. SQL is still highly popular among developers who prefer a more structured database compared to NoSQL databases, which is why SQL is often found within popular database migration tools. To start, we will show you how to create and read tables. Then we will move on to more advanced topics, such as joins and subqueries.
Creating and Reading Tables
The simplest thing you can do with SQL is create and read tables. To create a table, you use the CREATE TABLE statement. Here ‘s an example:
CREATE TABLE employees ( employee_id INTEGER NOT NULL AUTO_INCREMENT, first_name VARCHAR(50), last_name VARCHAR(50), email VARCHAR(100), position VARCHAR(50), salary DECIMAL(10, 2)) ENGINE=InnoDB;
This statement will create a table called employees that contains the following columns: employee_id, first_name, last_name, email, position, salary. The column employee_id will be an integer that is automatically incremented, first_name will be a 50-character string, last_name will be a 50-character string, email will be a 100-character string, position will be a 50-character string, and salary will be a 10-character string with 2 decimal places. The table will use the InnoDB engine.
To read data from a table, you use the SELECT statement. Here ‘s an example:
SELECT * FROM employees;
This statement will return all the data in the employees table.
Updating tables
You can also update tables with SQL. To update a table, you use the UPDATE statement. Here ‘s an example:
UPDATE employees SET email='jane.doe@example.com' WHERE employee_id=5;
This statement will update the email column for the employee with ID 5 to “jane.doe@example.com”.
Deleting Tables
You can also delete tables with SQL. To delete a table, you use the DROP TABLE statement.
Here ‘s an example:
DROP TABLE employees;
This statement will delete the employees table.
Joining Tables
Now that we have covered the basics of SQL, let ‘s move onto more advanced topics. One of the most powerful features of SQL is its ability to join tables together. Joining tables allows you to combine data from multiple tables into a single table.
There are many different types of joins, but the most common type is the inner join. An inner join is a join where the data in both tables is combined. Here’s an example:
SELECT * FROM employees INNER JOIN addresses ON employees.employee_id=addresses.employee_id;
This statement will return all the data from the employees and addresses tables, including any data that matches on the employee_id column.
Subqueries
A subquery is a query that is embedded within another query. It can be used to filter data or to calculate values. Here’s an example:
SELECT position, (SELECT salary FROM employees WHERE position='Sales Representative') AS salary FROM employees;
This statement will return the position and salary for all employees, but only for employees who have the position of “Sales Representative”. The subquery is used to calculate the salary for each employee.
Window functions
Window functions are a powerful feature of SQL that allow you to perform calculations on data that is grouped or sorted. Here’s an example:
SELECT first_name, last_name, SUM(salary) OVER (PARTITION BY first_name) AS total_salary FROM employees;
This statement will return the first name, last name, and total salary for each employee. The SUM function is used to calculate the total salary for each employee. And the OVER clause is used to group and sort the data.
What is SQL injection?
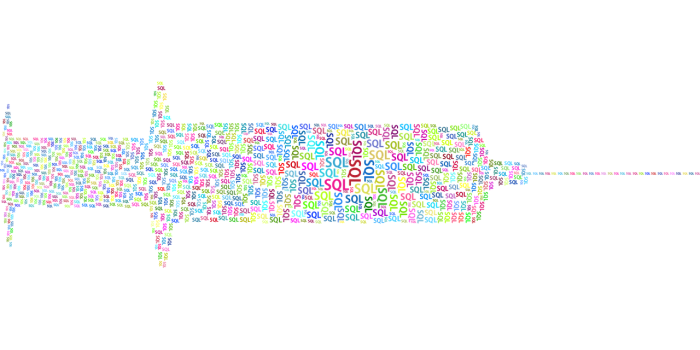
SQL injection is a security vulnerability that can be exploited when entering data into a website or application. It allows an attacker to inject SQL commands into an input field, which can be used to steal data or execute malicious actions.
SQL injection is a serious vulnerability as attackers can gain a sitewide control if they obtain administrative login credentials via the attack. If the database is not secured, and inputs aren’t tested properly, then the web server is vulnerable to an SQL attack.
How To Prevent SQL Injection Attack?
Countering SQL injections is possible by ensuring to take some precautionary measures. The cure for an attack could be a more challenging task than taking steps to prevent an attack. This is why QA Software Testers perform different types of software testing to build a foolproof codebase. Here are some of the most common and effective ways to prevent an SQL attack.
Prepared Statement
To prevent an SQL injection attack, parameterized SQL queries can be created for only allowing a fixed user input for successful execution of a command. This would prevent attackers from inserting a malicious script by preventing it from running as a modified SQL command.
Whatever the attacker inserts, it will be seen as a value for the prepared statement, which would restrict the attacker from accessing the database directly. This would allow you to improve your database security against SQL injections.
The following code is an example of a prepared statement in Java that uses the person’s name as input. The input is first converted into a string and then passed as a parameter to the query.
String name = "John"; String query = "SELECT * FROM person WHERE name=?"; PreparedStatement ps = connection.prepareStatement(query); ps.setString(1, name);
Stored Procedure
A stored procedure is a set of SQL commands that can be executed together. It is a good idea to use stored procedures because they increase the security of your code and also improve performance by reducing network traffic. Stored procedures are also harder for attackers to manipulate since they are not as visible as regular SQL queries.
The following code is an example of how to create a stored procedure in MySQL. The procedure takes an input of the person’s name and outputs the person’s information.
CREATE PROCEDURE getPerson(IN name VARCHAR(255)) BEGIN SELECT * FROM person WHERE name=name; END
Input Validation
Catching and fixing any malformed user input before sending it to the database server is another way of preventing SQL injection. User input should always be checked for type, length, syntax, and range. Invalid input should either be rejected or sanitized to prevent further damage.
To maintain a solid input validation procedure, the web developer should utilize regular expressions as whitelists for structured data (i.e name, age, address, survey response) to ensure strong input validation. This approach is far more efficient than blacklisting unauthorized commands. The whitelist protects your database against malicious commands.
This creates a security layer that verifies whether each input matches with the whitelisted commands or not. By using such a barrier for scanning inputs before they are allowed to run as a command, we prevent the modified inputs from executing malicious activity.
In this example, we have created a list of approved input commands. The user’s input is then checked against this list before it is allowed to run as a command.
Approved Input Commands:
SELECT, INSERT, UPDATE, DELETE
User Input:
SELECT * FROM users WHERE id=1;
Result:
The user’s input is valid and the command is executed.
An example where the result prompts invalid command:
User Input:
SELECT * FROM users WHERE id=1; DROP TABLE users;
Result:
The user’s input is invalid and the command is not executed.
In this way, we can validate user input and prevent SQL injection attacks.
Avoiding Admin Privilege:
SQL attacks can also be prevented if separate sign-in accounts are created for the applications and for access to the database. Keeping different credentials for the different applications used to access the database and not connecting your superuser account with it, can reduce the risk of severe damage to your database.
Example:
User ‘A’ has admin privileges to the database. User ‘B’ only has read access to the database through an application.
If user ‘A’ clicks on a malicious link, the attacker only gets read access to the database. However, if user ‘B’ clicks on the same malicious link, the attacker gets full admin access to the database as user ‘B’ has access to the database through an application.
Enforcing Least Privilege:
Least privilege is the security practice of granting users only the permissions they need to perform their work. By keeping the principle of least privilege in mind, you can further harden your database against SQL injection attacks.
Example:
User ‘A’ is a member of the ‘Admins’ group. The Admins group has full access to the database. User ‘B’ is a member of the ‘Users’ group. The Users group only has read access to the database.
If user ‘A’ clicks on a malicious link, the attacker gets full access to the database. However, if user ‘B’ clicks on the same malicious link, the attacker only gets read access to the database.
Web Application Firewalls
A Web Application Firewall, often known as WAF, is another option for preventing SQL injection. A WAF uses a set of regulations on HTTP communication. A proxy protects the client; a WAF, on the other hand, secures servers. It might be an appliance-based or software-based protection that adds security features to a Web application.
The advantage of utilizing a software-based WAF is that it may be readily integrated into the server or application. There is no need to modify the Web infrastructure in order to take advantage of this feature. The embedded WAFs also work properly, since they operate within the Web and monitor HTTP communications. Appliance-based firewalls are more efficient at monitoring multiple Web applications because the server resources are less utilized.
SQL Skills, and Career Opportunities
Many professions may benefit from SQL skills, not just those in the database administrator, data warehouse architect, database programmer, and other roles that require knowledge of SQL.
There are multiple ways in which experience of SQL can benefit professionals:
- SQL is a language for manipulating data. Data scientists that work with hundreds of tables spread across several databases need considerable SQL expertise.
- For working with data warehouses and structured databases, business intelligence analysts must possess strong SQL abilities.
- Experience with SQL is necessary for data analysts.
- It’s necessary to possess a good understanding of SQL as a cloud engineer.
- Owing to the popularity of data science and the growing demand for databases to collect huge amounts of information, a Financial Analyst can massively benefit from working on his SQL skills that combine both database design as well as data science. This is what makes the knowledge of SQL so essential for financial analysis
Conclusion
Given its longevity and importance to so many different applications, learning SQL can be very useful as a way to advance your career if you are interested and dedicated. There are several resources available to assist you in learning, so be sure to keep reading!
FAQs
Is SQL easy to learn? SQL can be generally regarded as an easy to learn language, and you can learn it in a span of two to three weeks, but only if you have working knowledge of some other programming language. In case, you’re starting from scratch as a beginner, it would be much more challenging and time consuming. |
Is SQL similar to Python? There are many differences between SQL and Python, however, the key difference is that SQL is used for accessing and extracting data from database, whereas Python is used to manipulate and analyze data through various data processing methods, such as regression testing and time series tests. |
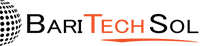
Empower your digital initiatives with BariTechSol, a premier custom software development company. Our skilled team tailors cutting-edge solutions to your unique needs. Elevate your tech experience and stay ahead in the digital realm. Partner with BaritechSol and code the success of your next big idea.